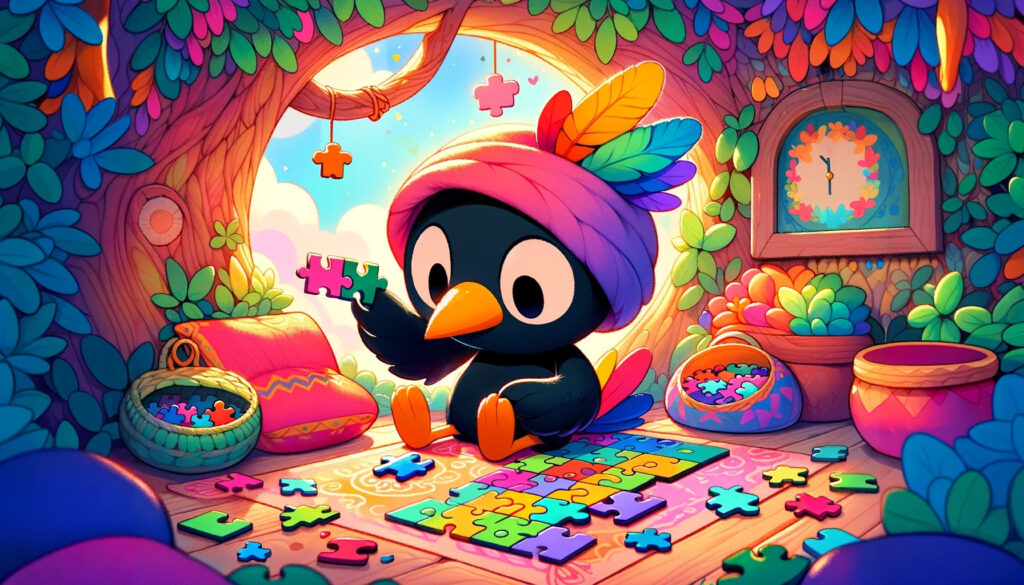
- Musical Intro
- Introduction
- Arithmetic operators: + , – , * , / , // , %
- Assignment Operators: = (and not ==)
- Comparison operators: == , > , >= , <= , < , !=
- Compound assignment operators: += , -= , *= , /=
- Rounding operators: round, flour, celling
- Exponentiation operators: 2Β³ , pow(2,3) , 2**3 , β9 , sqrt(9) , 9**.5
- Logical operators: & , |
- Data consistency operators: Math.abs()
- Other advanced operators
- Conclusion
- Using AI
Musical Intro
Introduction
In programming, operators are symbols that perform operations like mathematical calculations.
Now you need to make a choice, how you want to learn it:
- You can use AI to teach you about the different operators. Then you can learn, by your own exploration and in any programming language (scroll down to Using AI)
- You can use the result I got, from using AI. Then you can rely on my experience to filter it.(keep on reading)
- You can also do both in any order :-)
Arithmetic operators: + , – , * , / , // , %
Arithmetic operators are used to perform mathematical operations on numerical values.
It is written in Python, but you can use AI to translate it to other languages.
# Given two numbers
num1 = 10
num2 = 3
# When using arithmetic operators
addition = num1 + num2
subtraction = num1 - num2
multiplication = num1 * num2
division = num1 / num2
divisionRoundDown num1 // num2
modulus = num1 % num2 # Returns the remainder of the division
# Then the results should be as expected
print(f"Addition: {addition}")
assert addition == 10+3
print(f"Subtraction: {subtraction}")
assert subtraction== 10-3
print(f"Multiplication: {multiplication}")
assert multiplication== 10*3
print(f"Division: {division}")
assert division == 10/3
print(f"divisionRoundDown: {divisionRoundDown}")
assert divisionRoundDown== 10//3 # is 3.333... rounded down is 3
print(f"Modulus: {modulus}")
assert modulus== 10%3 # 10 will be split into three 3's and 1 will remain
The result will be:
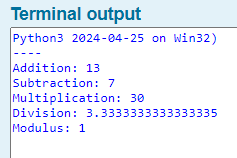
In case you wonder over why 10/3 equals 3.3333333333333335, it is because of a floating error (lack of precision). We will go into it on a later stage.
Challenge
# given we want to arrange team activities for attendies
menAttendees = 27
womenAttendees = 25
childrenAttendees = 11
groupSize = 5
# when we calculate the number of total attendees
totalAttendees = # please fill this out
# then it must match the expected result
print(f"Total attendees: {totalAttendees}")
assert totalAttendees == 63
# when we calculate the number of groups
numbeOfGroups = # please fill this out
# then it must match the expected result
print(f"Number of groups: {numbeOfGroups}")
assert numbeOfGroups == 12
# when we calculate the number of people without a groups
attendeesWithoutAGroup = # please fill this out
# then it must match the expected result
print(f"Attendeeswithout a group: {attendeesWithoutAGroup}")
assert attendeesWithoutAGroup == 3
print("All calculations are correct!")
Hint
use + for totalAttendees
use // for numberOfGroups
use % for attendeesWithoutAGroup
Result
# when we calculate the number of total attendees
totalAttendees = menAttendees + womenAttendees + childrenAttendees
# when we calculate the number of groups
numbeOfGroups = totalAttendees // groupSize
# when we calculate the number of people without a groups
attendeesWithoutAGroup = totalAttendees % groupSize
Assignment Operators: = (and not ==)
When we use = then we assign a value to a variable.
When we use == then we verify if something is equal or not. The result is either true or false
# When we assign three values to three variables with an =
a = 1
b = 1
c = 2
# Then we can verify the values with an ==
print(a==b)
print(a==c)
And the result will be:
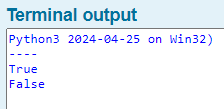
Challenge
# please fix the followin code, so it works
a = 1
b == 1
assert a=b
print("you did it!")
Hint
look for = where we need to assign a value
look for == where we need to verify a value
Result
# please fix the followin code, so it works
a = 1
b = 1
assert a==b
print("you did it!")
Comparison operators: == , > , >= , <= , < , !=
We can also verify other than just equal
print("greater than >")
print(f"4>3 -> {4>3}")
print(f"3>3 -> {3>3}")
print("----")
print("greater equal than >=")
print(f"4>=3 -> {4>=3}")
print(f"3>=3 -> {3>=3}")
print(f"2>=3 -> {2>=3}")
print("----")
print("lesser equal than <=")
print(f"4<=3 -> {4<=3}")
print(f"3<=3 -> {3<=3}")
print(f"2<=3 -> {2<=3}")
print("----")
print("lesser than <")
print(f"3<3 -> {3<3}")
print(f"2<3 -> {2<3}")
print("----")
print("not equal !=")
print(f"3!=4 -> {3!=4}")
print(f"3!=3 -> {3!=3}")
print(f"4!=3 -> {4!=3}")

Challenge
# please fix the followin code, so it works
assert 3>4
assert 4<4
assert 5<4
assert 5=="abc"
print("you did it!")
Hint
use >, <, ==, and !=
Result
# please fix the followin code, so it works
assert 3<4
assert 4==4
assert 5>4
assert 5!="abc"
print("you did it!")
Compound assignment operators: += , -= , *= , /=
To make code shorter, we can use:
# When we have a number variable
number = 1
print(number)
# Then we want to increase a number by 3 we can write:
number = number + 3
print(number)
assert number == 4
# Or
number += 3
print(number)
assert number == 7

# When we have a number variable
number = 5
print(number)
# Then we want to decrease a number by 3 we can write:
number = number - 3
print(number)
assert number == 2
# Or
number -= 3
print(number)
assert number == -1

# When we have a number variable
number = 1
print(number)
# Then we want to multiply a number by 3 we can write:
number = number * 3
print(number)
assert number == 3
# Or
number *= 3
print(number)
assert number == 9
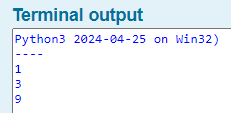
# When we have a number variable
number = 8
print(number)
# Then we want to multiply a number by 2 we can write:
number = number / 2
print(number)
assert number == 4
# Or
number /= 2
print(number)
assert number == 2

Challenge
# refactor the following code to use +=, -=, *=, /=
number1 = 3
number1 = number1 + 3
number1 = number1 - 2
number1 = number1 * 3
number1 = number1 / 2
assert number1 == 6
print("you did it!")
Result
# refactor the following code to use +=, -=, *=, /=
number1 = 3
number1 += 3
number1 -= 2
number1 *= 3
number1 /= 2
assert number1 == 6
print("you did it!")
Rounding operators: round, flour, celling
A number can be rounded to a specific decimal by using a Math library:
# given we have a number variable
number1 = 1.4999999
number2 = 1.5000000
# when we round it in multiple ways:
roundedDown = round(number1)
roundedUp = round(number2)
# then it will be rounded up or down, based on if it's half way or not.
print(f"roundedDown: {number1}->{roundedDown}")
assert roundedDown == 1
print(f"roundedUp: {number2}->{roundedUp}")
assert roundedUp == 2
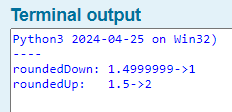
To always round down we can use math.floor():
import math
# given we have a number variable
number1 = 1.0000000
number2 = 1.9999999
number3 = 2.0000000
# when we round it in multiple ways:
floor1 = math.floor(number1)
floor2 = math.floor(number2)
floor3 = math.floor(number3)
# then it will be rounded up or down, based on if it's half way or not.
print(f" {number1}-> {floor1}")
assert floor1 == 1
print(f" {number2}-> {floor2}")
assert floor2 == 1
print(f" {number3}-> {floor3}")
assert floor3 == 2
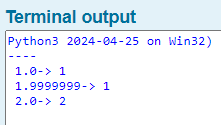
To always round down we can use math.ceil():
import math
# given we have a number variable
number1 = 1.0000000
number2 = 1.0000001
number3 = 1.9999999
# when we round it in multiple ways:
floor1 = math.ceil(number1)
floor2 = math.ceil(number2)
floor3 = math.ceil(number3)
# then it will be rounded up or down, based on if it's half way or not.
print(f" {number1}-> {floor1}")
assert floor1 == 1
print(f" {number2}-> {floor2}")
assert floor2 == 2
print(f" {number3}-> {floor3}")
assert floor3 == 2

Challenge
import math
# given you have the following numbers
number1a = # insert a correct value here
number1b = # insert a correct value here
number2a = # insert a correct value here
number2b = # insert a correct value here
number3a = # insert a correct value here
number3b = # insert a correct value here
# when you apply math.round math.floor, and math.ceil:
rounded1a = math.round(number1a)
rounded1b = math.round(number1b)
rounded2a = math.floor(number2a)
rounded2b = math.floor(number2b)
rounded3a = math.ceil(number3a)
rounded3b = math.ceil(number3b)
# then you must get the expected values
assert rounded1a == 1, f"{number1a} -> {rounded1a}"
assert rounded1b == 2, f"{number1b} -> {rounded1b}"
assert rounded2a == 3, f"{number2a} -> {rounded2a}"
assert rounded2b == 4, f"{number2b} -> {rounded2b}"
assert rounded3a == 5, f"{number3a} -> {rounded3a}"
assert rounded3b == 6, f"{number3b} -> {rounded3b}"
print("you did it!")
Result
import math
# given you have the following numbers
number1a = 1.499999
number1b = 1.500000
number2a = 3.999999
number2b = 4.000000
number3a = 5.000000
number3b = 5.000001
# when you apply math.round math.floor, and math.ceil:
rounded1a = math.round(number1a)
rounded1b = math.round(number1b)
rounded2a = math.floor(number2a)
rounded2b = math.floor(number2b)
rounded3a = math.ceil(number3a)
rounded3b = math.ceil(number3b)
# then you must get the expected values
assert rounded1a == 1, f"{number1a} -> {rounded1a}"
assert rounded1b == 2, f"{number1b} -> {rounded1b}"
assert rounded2a == 3, f"{number2a} -> {rounded2a}"
assert rounded2b == 4, f"{number2b} -> {rounded2b}"
assert rounded3a == 5, f"{number3a} -> {rounded3a}"
assert rounded3b == 6, f"{number3b} -> {rounded3b}"
print("you did it!")
Exponentiation operators: 2Β³ , pow(2,3) , 2**3 , β9 , sqrt(9) , 9**.5
If we want write 3Β², which is 3*3 = 9
or 2Β³, which is 2*2*2 = 8
We can do that with math.pow()
import math
# when we user mat.pow() method
number1 = math.pow(2,3)
number2 = math.pow(3,2)
# and the ** can also be user
number3 = 2**3
# then we can see the power of the values
print(f" pow(2,3) = 2*2*2 -> {number1}")
assert number1 == 8
print(f" pow(3,2) = 3*3 -> {number2}")
assert number2 == 9
print(f" 2**3 = 2*2*2 -> {number3}")
assert number3 == 8
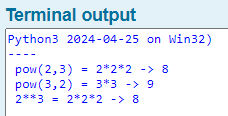
when we want the opposite of power we can either use math.sqrt() (square root) or fractions in math,pow()
import math
# when we user math.sqrt() method
number1 = math.sqrt(9)
# and we can use the math.power() with fractions
number2 = math.pow(9, 1/2)
# and we can use the math.power() with fractions
number3 = 9**(1/2)
# then we can see the power of the values
print(f" sqrt(9) -> {number1}")
assert number1 == 3
print(f" pow(9, 1/2) -> {number2}")
assert number2 == 3
print(f" 9**(1/2) -> {number3}")
assert number3 == 3

Challenge
import math
# change the numbers to make the math work:
result1 = math.pow(1,1)
result2 = math.sqrt(4)
result3 = 1**2
result4 = 4**(1/2)
result5 = 9**(.5)
# then you must get the expected values
assert result1 == 25, f"{result1} != 25"
assert result2 == 4, f"{result1} != 4"
assert result3 == 16, f"{result1} -!= 16"
assert result4 == 6, f"{result1} != 6"
assert result5 == 8, f"{result1} != 8"
print("you did it!")
Result
import math
# change the numbers to make the math work:
result1 = math.pow(5,2)
result2 = math.sqrt(16)
result3 = 2**4
result4 = 36**(1/2)
result5 = 64**(.5)
# then you must get the expected values
assert result1 == 25, f"{result1} != 25"
assert result2 == 4, f"{result1} != 4"
assert result3 == 16, f"{result1} -!= 16"
assert result4 == 6, f"{result1} != 6"
assert result5 == 8, f"{result1} != 8"
print("you did it!")
Logical operators: & , |
& and | is more about logic.
3 == 3 gives true, while 3==4 gives false
we can combine the the output with & and | operators.
Beware that in Python it is True and False, with UPPER CASE
In Groovy, JavaScript and C# it is true an false with lower case
In other languages it can be TRUE and FALSE.
The following gives an overview. It will make more sense with if-statements, that will come in a later lesson..
print("and &")
print(f"True & True -> {True & True}")
print(f"True & False -> {True & False}")
print(f"False & True -> {False & True}")
print(f"False & False -> {False & False}")
print("----")
print("and |")
print(f"True | True -> {True | True}")
print(f"True | False -> {True | False}")
print(f"False | True -> {False | True}")
print(f"False | False -> {False | False}")
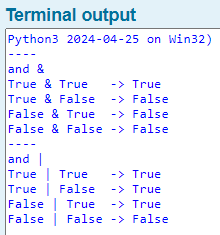
True and false can also be written as 1 (true) and 0 (false)
print("and &")
print(f"1 & 1 -> {1 & 1}")
print(f"1 & 0 -> {1 & 0}")
print(f"0 & 1 -> {0 & 1}")
print(f"0 & 0 -> {0 & 0}")
print("----")
print("and |")
print(f"1 | 1 -> {1 | 1}")
print(f"1 | 0 -> {1 | 0}")
print(f"0 | 1 -> {0 | 1}")
print(f"0 | 0 -> {0 | 0}")
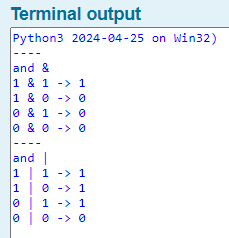
Data consistency operators: Math.abs()
Math.abs() makes all values positive.
import math
print(-5)
print(math.abs(-5))
print(math.abs(5))
print(5)
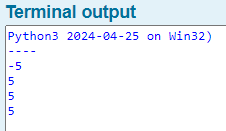
Challenge
import math
# given you have the following values
number1 = -2
number2 = -3
# when you add them together correctly
result = number1 + number2
# then you must get the expected values
assert number1 == -2, f"number1 must be -2, but is {number1}"
assert number2 == -3, f"number1 must be -3, but is {number2}"
assert result == 5, f"result must be 5, but is {result}"
print("you did it!")
Hint
Remember that math.abs(-3) is 3
Result
# when you add them together correctly
result = math.abs(number1) + math.abs(number2)
Other advanced operators
There are many other operators like: sin()
, cos()
, tan()
, asin()
, acos()
, atan()
, atan2()
, log()
, log10()
, log2()
, mean()
, median()
, variance()
, std()
, etc.
We will cover these in a later lesson.
Conclusion
Today, we embarked on an adventurous journey into the realm of operators, those magical symbols that enable us to manipulate data in programming.
We began by mastering arithmetic operators like addition, subtraction, multiplication, division, and modulus, empowering us to perform fundamental mathematical calculations effortlessly.
Transitioning to assignment operators, we learned to assign values to variables with “=“, and verify equality with “==“, streamlining our code for efficient data management.
We then ventured into comparison operators, including greater than (>), greater than or equal to (>=), lesser than (<), lesser than or equal to (<=), and not equal (!=), enabling us to make logical decisions based on variable comparisons.
To enhance code readability and efficiency, we embraced compound assignment operators like increment (+=), decrement (-=), multiply (*=), and divide (/=), simplifying variable modifications.
Delving deeper, we explored mathematical rounding techniques such as round(), math.floor(), and math.ceil(), ensuring precision in numerical calculations.
We harnessed the power of exponentiation with the ** operator and math.pow() function, facilitating easy calculation of powers and square roots math.sqrt().
Additionally, we harnessed logical operators like AND (&) and OR (|), enabling us to evaluate complex logical expressions with ease.
Ensuring data consistency operator, we utilized the Math.abs() function to transform negative numbers into positive ones, maintaining uniformity in data processing.
While we’ve only scratched the surface of operators in programming, today’s journey has provided us with a solid foundation to build upon. Armed with these newfound skills, we’re better equipped to tackle a wide array of computational tasks and navigate the diverse landscapes of programming with confidence.
Using AI
All this have been created with the cooperation with AI.
Often it can be more useful just to learn, what is needed in the moment, than to learn everything about a topic. This is where AI is perfect.
We can use the free ChatGPT 3.5 to learn about operators. You are welcome to try other and if you do, please write a comment!
Essential prompts can be:
When we don’t know the name of what we want to learn, then we can ask AI about it:
What do we call + - * and / in Python?

When we want to learn more about the topic
What other operators are there?
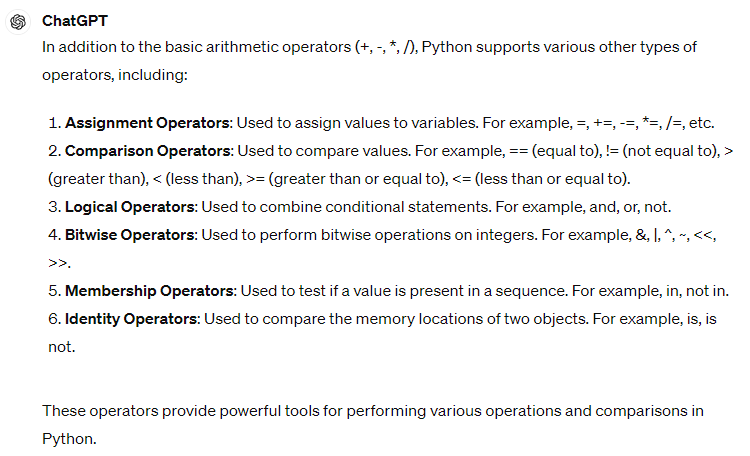
You can ask the same question twice and get more results:
Are there others?
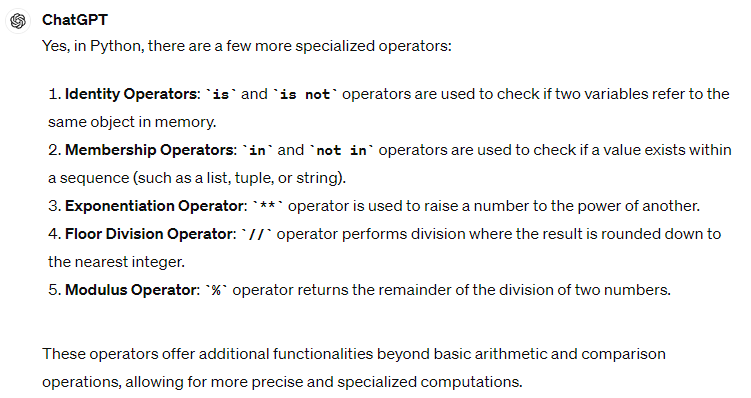
Then you can explore each operator with:
I would like to learn more about Floor Division Operator //.
Can you make a assignment for me to solve?
The assignment needs to be written in Python as comments.
Use only given, when, and then comments. No code.
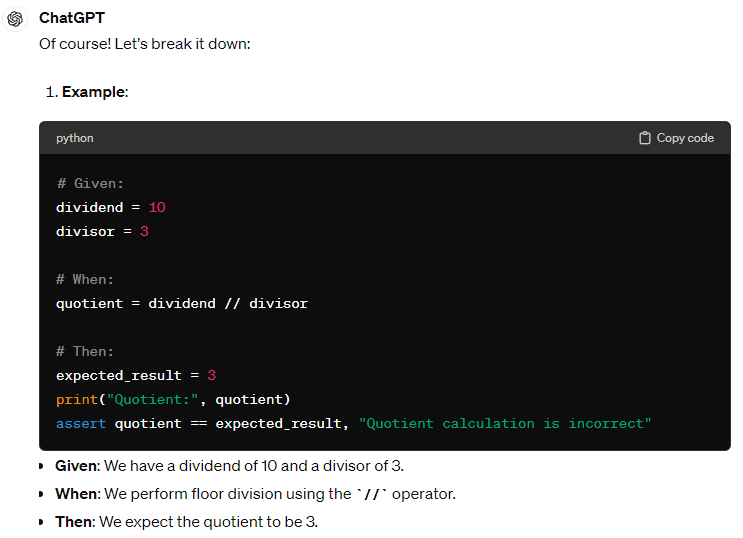
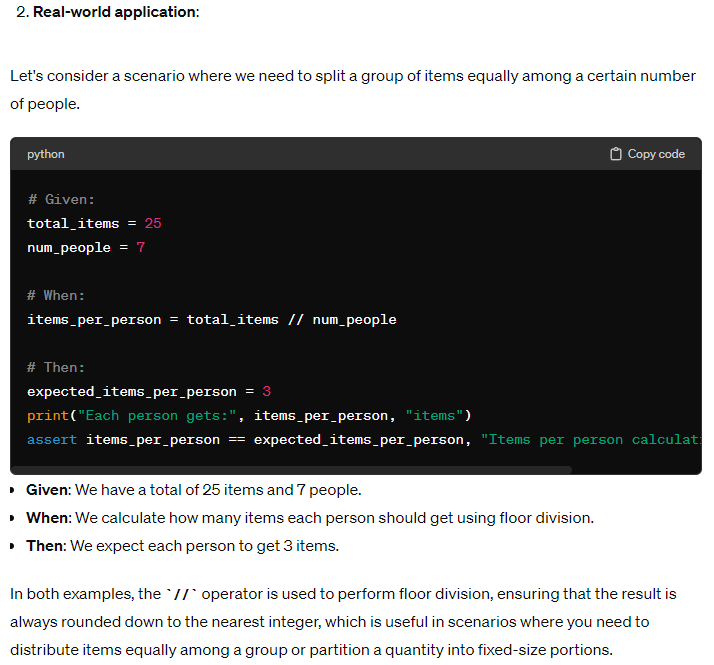
To get an assignment to learn from:
I would like to learn more about Floor Division Operator //.
Can you show me:
1. an example of how it works? (in python)
2. a real world application of it? (in python)
I want the code examples to be written with given, when, and then steps.
The then step needs to contain a print and assert (for manual and automatic verification)

And sometimes you might need to start a new session.
Sometimes the AI makes a mistake like writing Given, Then, When (wrong order) and sometimes starting a new session makes it go away.
And you can get AI to solve it for you:
Can you please solve this assignment for me in Python code?
--
# Given:
# Assume you are organizing a marathon event.
# You have a total of 152 registered participants.
# Each starting block can accommodate a maximum of 40 runners.
# When:
# Calculate the total number of starting blocks needed for the marathon.
# Use the floor division operator (//) to ensure that any partial block
# needed is rounded up to the nearest whole block.
# Then:
# Print the total number of starting blocks required for the marathon.
# Assert that the calculated total matches the expected value.
--
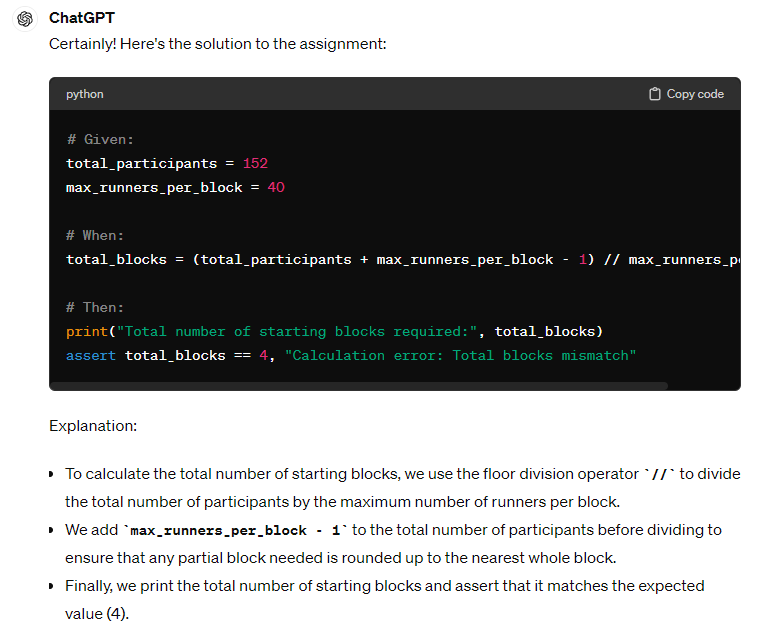
To be sure it works, you can always copy the code into your editor and see if it works!
Web editor: π» Python / π» JavaScript / π» Groovy / π» C#
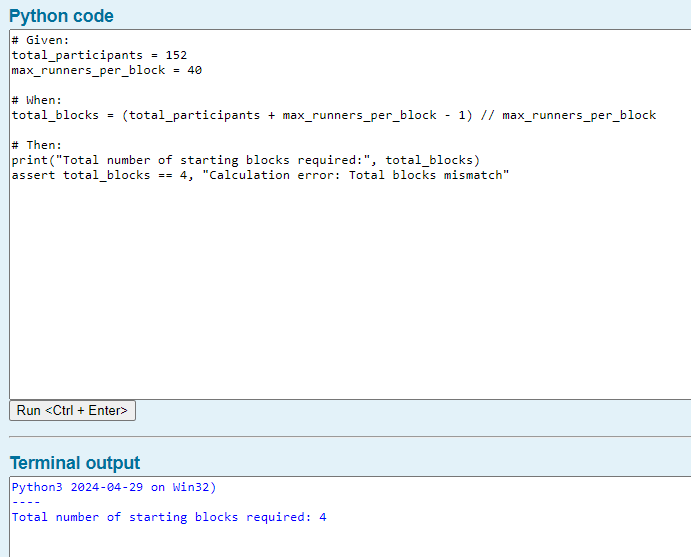
You can ask for a new assignment – as many times as you want.
You can make the AI solve it for you.
You can make the AI help you solve it.
You can solve it yourself.
Limitless learning with a 24/7 tutor!!!
Congratulations – Lesson complete!
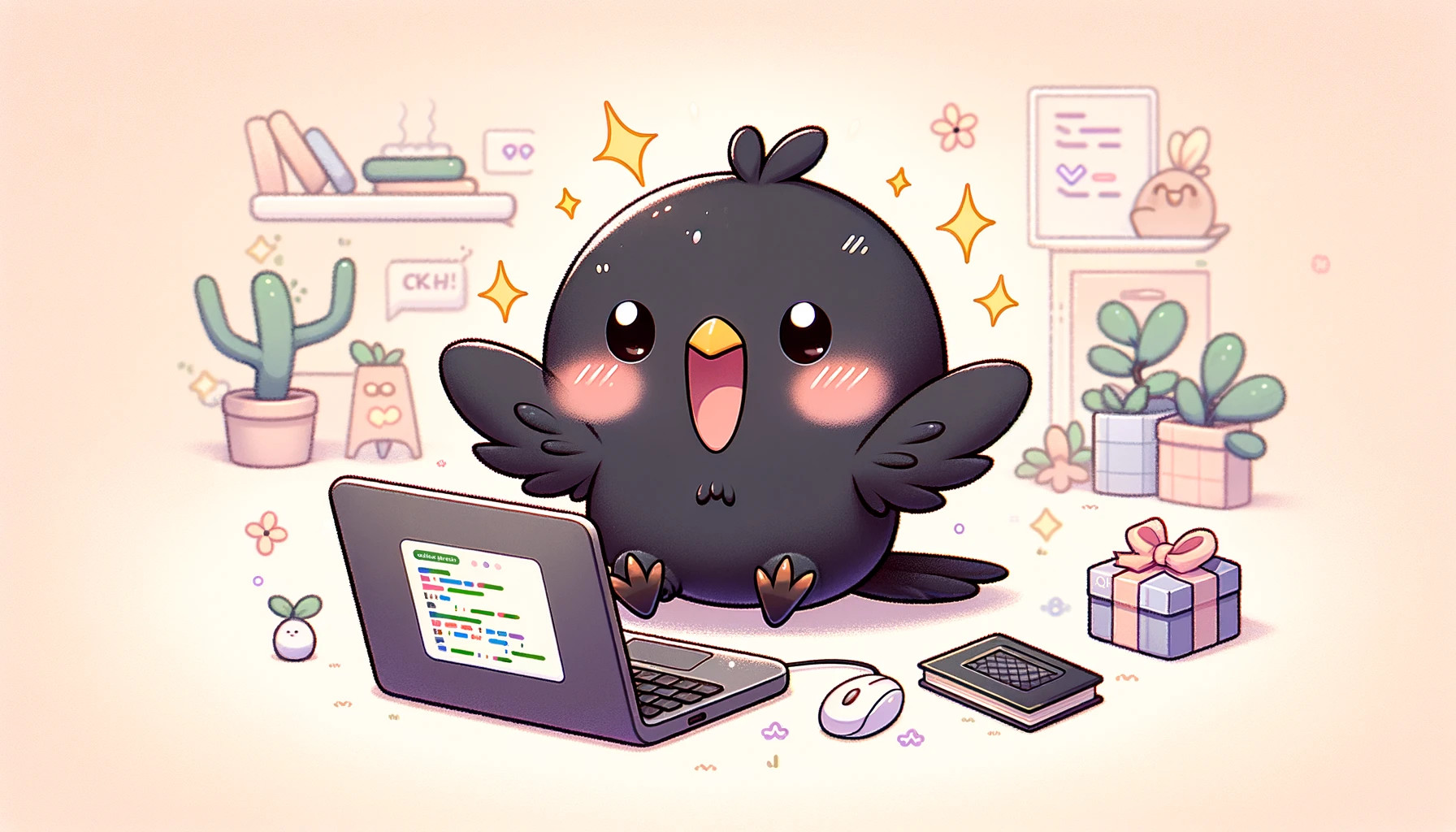