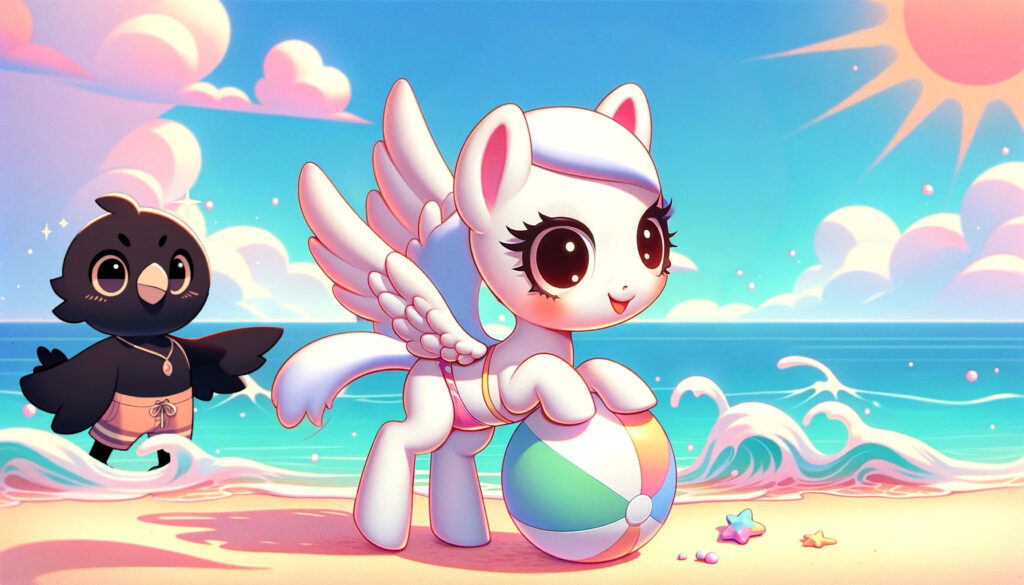
- Musical Intro
- Introduction
- Joining Strings Together (Adding / Concatenation)
- G-, F-, Template- and interpolated-strings
- Slicing
- Length of a string
- Replace parts of a string
- UPPER CASE and lower case
- Trim or strip
- Padding
- Escape sequences to make line breaks, tabulators, and quotes within quotes
- Advanced tools
- Conclusion
- Using AI
Musical Intro
Introduction
In programming, a “string” is simply text data – like words in a book or letters in your name.
Technically it is a variable, where you store text in it.
# when we have a text string
aTextString = "hello world"
# then we can print it to show it's content
print(aTextString)
Now you need to make a choice, how you want to learn it:
- You can use AI to teach you. Then you can learn, by your own exploration and in any programming language (scroll down to Using AI)
- You can use the result I got, from using AI. Then you can rely on my experience to filter it.(keep on reading)
- You can also do both in any order :-)
Joining Strings Together (Adding / Concatenation)
# given we have two texts
var1 = "hello"
var2 = "world"
# when we add them together
result = var1 + var2
# then they put together
print(result)
assert result == "helloworld"
Adding works differently with numbers:
# given we have two numbers
var1 = 1
var2 = 2
# when we add them together
result = var1 + var2
# then they give the sum
print(result)
assert result == 3
If we put the numbers as text (with quotation marks), then they will add as strings and not numbers:
# given we have two numbers
var1 = "1"
var2 = "2"
# when we add them together
result = var1 + var2
# then they put together (and not the sum)
print(result)
assert result == "12"
Also if you want to have a space between to words, that you add together, then you need to remember to also add a space:
# given we have two texts
text1 = "hello"
text2 = "world"
space = " " # the space
# when we add them together
result = text1+ space + text2
# then they put together
print(result)
assert result == "hello world"
Challenge, weather report
Try to write a code, where you
# given the weather is describe with the following parts:
city = "Praestoe"
temperature = "22°C"
# When we concatenate them to form a weather report
# (write your code here)
# Then the result should exactly match the expected report.
print(result)
assert result == "The current weather in Praestoe is 22°C"
Hint / Result
result = "The current weather in " + city + " is " + temperature
G-, F-, Template- and interpolated-strings
G-Strings are a special kind of strings that are used in Groovy. They have other names in other languages:
Language | Example | Name |
Python | f"text {parameter] text" | f-string |
JavaScript | `text ${parameter} text` | template string |
C# | $"text {parameter} text" | interpolated string |
Groovy | "text {parameter} text" | g-string |
They seem much more complex that a regular string, except they make our lives much easier.
Which one of these text addition do you find easiest to use?
# example 1 (the regular string)
result = "Mr. "+ name
# example 2 (the f-string)
result = f"Mr. {name}"
When we write a f in front of a “
Then we can just add { } with a variable name inside the text.
This makes it much easier with much more complicated strings, where we can use spaces inside the string, instead of adding extra spaces or adding them as variables:
# example 1 (the regular string)
result = title + " " + firstName + " " + lastName
# example 2 (the regular string with space variables)
result = title + space + firstName + space + lastName
# example 3 (the f-string)
result = f"{title} {firstName} {lastName}"
Challenge, F-weather
Rewrite the previous challenge by using f-strings
# given the weather is describe with the following parts:
city = "Praestoe"
temperature = "22°C"
# When we concatenate them to form a weather report
# (write your code here)
# Then the result should exactly match the expected report.
print(result)
assert result == "The current weather in Praestoe is 22°C"
Hint / Result
result = f"The current weather in {city} is {temperature}"
Slicing
Since we can add strings together, then we can also slice them up into smaller parts and only keep the important part.
# given we have a text string
greeting = "Hello world"
# when we slice off the first letter
without_first = greeting[1:]
# then we only have the rest left
print(without_first)
assert without_first == "ello world"
And the same can be done on the other side of the string:
# given we have a text string
greeting = "Hello world"
# when we slice of the 2 last letters
without_last = greeting[:-2]
# then we only have the rest left
print(without_first)
assert without_first == "hello wor"
We use negative numbers, to count letters from the back of the string
We use positive numbers, to count letters from the front of the string
Challenge, slicing the world
# given we have a text string
greeting = "12345"
# when the ? is replaced with a positive number
result_1_1 = greeting[?:]
result_1_2 = greeting[:?]
result_1_3 = greeting[?:?]
# and the ? is replaced with a negative number
result_2_1 = greeting[:-?]
result_2_2 = greeting[-?:]
result_2_3 = greeting[-?:-?]
# then the strings are sliced to
assert result_1_1 == "2345"
assert result_1_2 == "12"
assert result_1_3 == "2"
assert result_2_1== "1234"
assert result_2_2== "45"
assert result_2_3== "4"
Hint / Result
result_1_1 = greeting[?:] # [1:] from after letter 1
result_1_2 = greeting[:?] # [:2] until after letter 2
result_1_3 = greeting[?:?] # [1:2] from after letter 1 until after letter 2
result_2_1 = greeting[:?] # [:-1] until before letter 1 from behind
result_2_2 = greeting[?:] # [-2:] from before letter 2 from behind
result_2_3 = greeting[?:?] # [-2:-1] from before letter 2 from behind until
result_3_1 = greeting[?:?] # [1:-2]from after letter 1 until before letter -2 from behind
result_3_1 = greeting[?:?] # [-3:1]from after letter 1 until before letter -2 from behind
Length of a string
We can also measure the length of a string, which can be important if we want a password to have a minimum length.
# when we have two passwords
password1 = "12345678"
password2 = "1234567"
# then password1 is long enough (this will pass)
assert len(password1) >=8, "error: password 1 not long enough"
# and password2 is not long enough (this will fail)
assert len(password2) >=8, "error: password 2 not long enough"
Replace parts of a string
When we have a string, then we can replace a part of it with something else.
# given we have a greeting
greeting = "Hello Jan Wallace"
# when we replace the name
greeting = greeting.replace("Jan", "Peter")
# then the complete greeting will change
print(greeting)
assert greeting == "Hello Peter Wallace"
We simply write the word or letters we want to replace, and the second word is what we want to replace it with.
Challenge, remove/replace a name
# given we have a letter, where a person is named
letter = "Hello Jan Wallace. We want to inform you, that your name Jan Wallace, will now be annonymized in the whole string."
# when we replace "Jan Wallace" with "J"
# write your own code here
letter = letter .replace("Jan Wallace", "J")
# then all
print(letter )
assert letter == "Hello J. We want to inform you, that your name J, will now be annonymized in the whole string."
Hint / Result
greeting = greeting.replace("Jan Wallace", "J")
UPPER CASE and lower case
Computers are always sensitive with UPPER CASE and lower case characters.
When we write a variable name with an upper case letter, then we need to remember that upper case letter.
# when we have a variable called fullName
fullName = "Jan Wallace"
# then we need to use the capital N otherwise
print(fullName)
# and if we don't use the capital N, then it will fail
print(fullname)
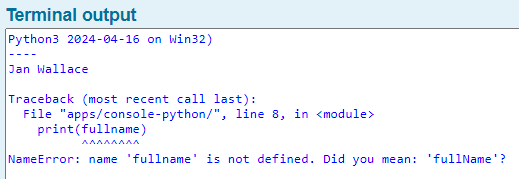
Sometimes we make it easier for the users to only use lower case letters, like we do with emails. The following emails are the same “spam@bartek.dk”, “SPAM@BARTEK.DK” and “SpAm@BaRtEk.Dk”.
To control the upper or lower case we can use the a commando:
# when we have 3 emails
email1 = "spam@bartek.dk"
email2 = "SPAM@BARTEK.DK"
email3 = "SpAm@BaRtEk.Dk"
# then we can turn all of them into the same email with lower case
print(email1.lower())
print(email2.lower())
print(email3.lower())
assert email1.lower() == "spam@bartek.dk"
assert email2.lower() == "spam@bartek.dk"
assert email3.lower() == "spam@bartek.dk"
# and we can do it with UPPER CASE too
print(email1.upper())
print(email2.upper())
print(email3.upper())
assert email1.upper() == "SPAM@BARTEK.DK"
assert email2.upper() == "SPAM@BARTEK.DK"
assert email3.upper() == "SPAM@BARTEK.DK"
Challenge, split and uppercase and add
We want the following 3 names to start with a uppercase letter and the rest be lower case. You will have to split your string make the first letter capital and add the new letter to the rest of the name.
# given we have 3 names
name= "eLISAbeTH"
# when we split it into first letter and the rest
# (write your code here)
# and make the first letter upper case
# (write your code here)
# and the rest lower case
# (write your code here)
# and add them all together (You may also use an f-string)
# (write your code here)
# then our name will start with an upper case letter and the rest will be lower case letters
print(name)
assert name == "Elisabeth"
Hint / Result
# given we have 3 names
name= "eLISAbeTH"
# when we split it into first letter and the rest
firstLetter = name[:1]
restOfName = name[1:]
# and make the first letter upper case
firstLetter = firstLetter.upper()
# and the rest lower case
restOfName = restOfName.lower()
# and add them all together (You may also use an f-string)
name = f"{firstLetter}{restOfName}"
# then our name will start with an upper case letter and the rest will be lower case letters
print(name)
assert name == "Elisabeth"
Trim or strip
Sometimes Strings are written with spaces before and after the text: ” hello world “
The easiest way to remove the spaces is to use the command strip() in Python, trim() in JavaScript and Groovy, and Trim() in C#.
# Given the original text with leading and trailing space
originalText = " hello world "
print(f"original: ${originalText }")
# When we apply strip in it's 3 variations:
text1 = originalText.lstrip()
text2 = originalText.rstrip()
text3 = originalText.strip()
# Then text1 should be with no leading whitespace
print(f"lstrip: |{text1}|")
assert text1 == "hello world "
# And text2 should be with no trailing whitespace
print(f"rstrip: |{text2}|")
assert text2 == " hello world"
# And text3 should be with no leading or trailing whitespace
print(f"strip: |{text3}|")
assert text3 == "hello world"
Padding
To add spaces, or any other symbol, before a text can be done with rjust() in Python, padStart() in JavaScript, padLeft() in Groovy, PadLeft() in C#.
To add spaces, or any other symbol, after a text can be done with ljust() in Python, padEn() in JavaScript, padRight() in Groovy, PadRight() in C#.
# Given product names and prices
product1 = "Orange"
price1 = "25 EUR"
product2 = "Computer"
price2 = "1000 EUR"
# When combining product names and prices with normal addition
productText1a = product1 + " " + price1
productText2a = product2 + " " + price2
# Then the product text is not formatting in a pretty way
print(productText1a)
print(productText2a)
print("--------- ---------")
# When aligning product names with spaces as padding
productText1b = product1.ljust(10, " ") + price1.rjust(10, " ")
productText2b = product2.ljust(10, " ") + price2.rjust(10, " ")
# Then the product text should have a pretty formatting
print(productText1b)
print(productText2b)
print("--------- ---------")
# When aligning product names with dots as padding
productText1c = product1.ljust(10, ".") + price1.rjust(10, ".")
productText2c = product2.ljust(10, ".") + price2.rjust(10, ".")
# Then the product text should have even prettier formatting
print(productText1c)
print(productText2c)
print("--------- ---------")
Which will give the following output:
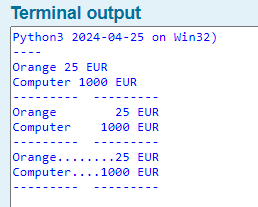
Escape sequences to make line breaks, tabulators, and quotes within quotes
To have a line break in a string, then a \n can be put into it. Because we can’t write:
print("line 1
line 2")
# creates an error
To have a tab a \t can be use, even though we sometimes can add tabs, but not always. Sometimes they are added as 2 or 4 spaces instead of a tab:
print("word 1 word 2")
# creates an error
To have a quote within a quote requires an \”, because we can’t write the following without creating an error:
print("word 1 "word 2" word 3")
# creates an error
And because we use \ to write special characters, we need to write \\ to tell the computer we want to write an \, otherwise it will expect an special character.
To write it correctly please do:
print("----linebreak----")
print("line 1\nline 2")
print("----tab----")
print("word 1\tword 2")
print("----quoate----")
print("word 1\"word 2\"word 3")
print("----backslash----")
print("before\\after")
and it gives the following output:

Advanced tools
There are even more tools like Regex, but we need to learn more like Arrays, indexing, functions, etc. We will come back to those later.
Conclusion
Today, we took a fun dive into the colorful world of strings, discovering that strings are like little containers for text—holding everything from single words to lengthy paragraphs.
We kicked things off by learning how to add strings together, transforming “hello” and “world” into “hello world.”
We then looked into f-strings (Python), G-strings (Groovy), template-strings (JavaScript) and interpolated strings (C#).
We also got crafty with slicing strings, which is a bit like sculpting: you chisel away the parts you don’t need or shuffle them around to create something entirely new.
And we played around with replacing a word in a string with another word. Quite fancy when you want to anonymize a text.
We also looked into the length of strings. It’s like having a tiny ruler at your fingertips, perfect for checking the length of a text, ensuring passwords meet security standards, or just satisfying your curiosity about how much you’ve actually typed.
We played with to uppercase and lowercase, which helps keep everything looking neat and tidy, no matter how chaotic the input might be.
We ended with a fun exercise where we had to combine all what we learned together, by making “eLISAbeTH “into “Elisabeth”
We looked into trim/strip to remove spaces.
Padding to add spaces and other symbols.
Escape sequences such as line breaks, tabulators, and quotes within quotes.
By now, you should feel pretty equipped to handle text in your programming projects. Whether you’re crafting messages, tidying up data, or just indulging your inner nerd, the skills you’ve embraced today are essential tools in your programming kit. Let’s keep that inner child curious and eager to learn more as we continue exploring the vast and vibrant world of coding!
Using AI
All this have been created with the cooperation with AI.
Often it can be more useful just to learn, what is needed in the moment, than to learn everything about a topic. This is where AI is perfect.
We can use the free ChatGPT 3.5 to learn about operators. You are welcome to try other and if you do, please write a comment!
Essential prompts can be:
Learning about Strings
I would like to learn about Strings (text) in Python.
What things are critical to learn?
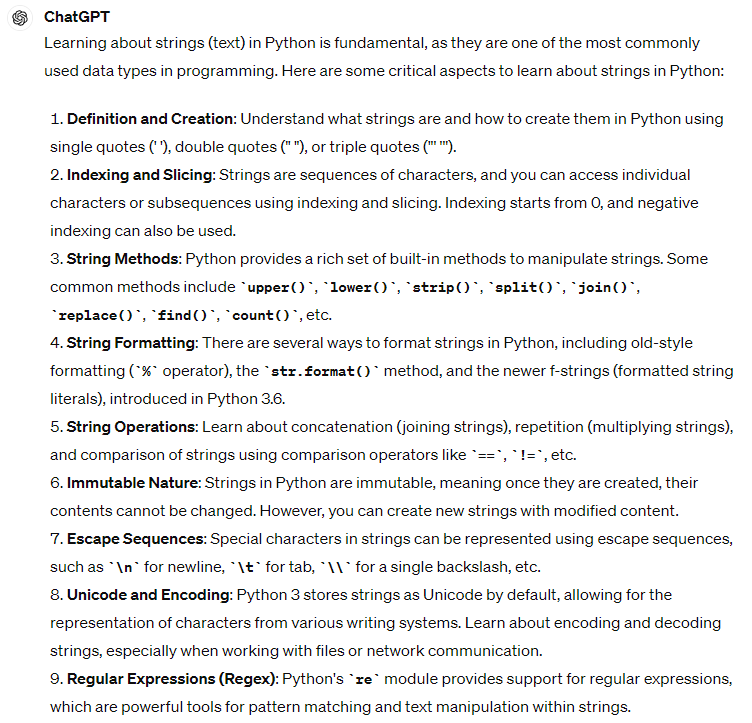
When we want to learn more about the topic
I would like to learn more about Escape Sequences, what should I be able to do?
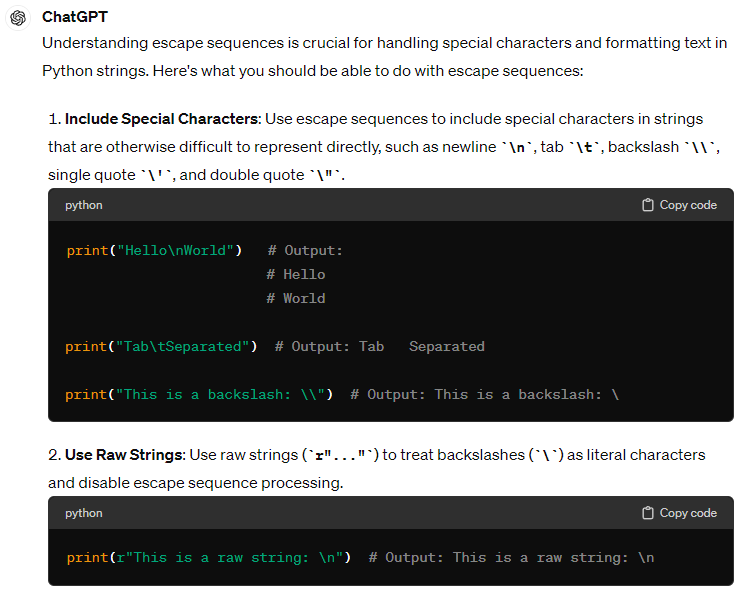
It answered much more, but there is no need to show it all, because the amount of data can be overwhelming: (Multiline Strings, Unicode Character, Escape Sequence as Literal, Control Characters, Hexadecimal and Octal Escapes)
Take one of them and go further into it.
Then you can explore each theme with:
I would like to learn more about Special Characters such as '\n', '\t', and '\\'.
Can you show me:
1. an example of how it works? (in python)
2. a real world application of it? (in python)
I want the code examples to be written with given, when, and then steps.
The then step needs to contain a print and assert (for manual and automatic verification)
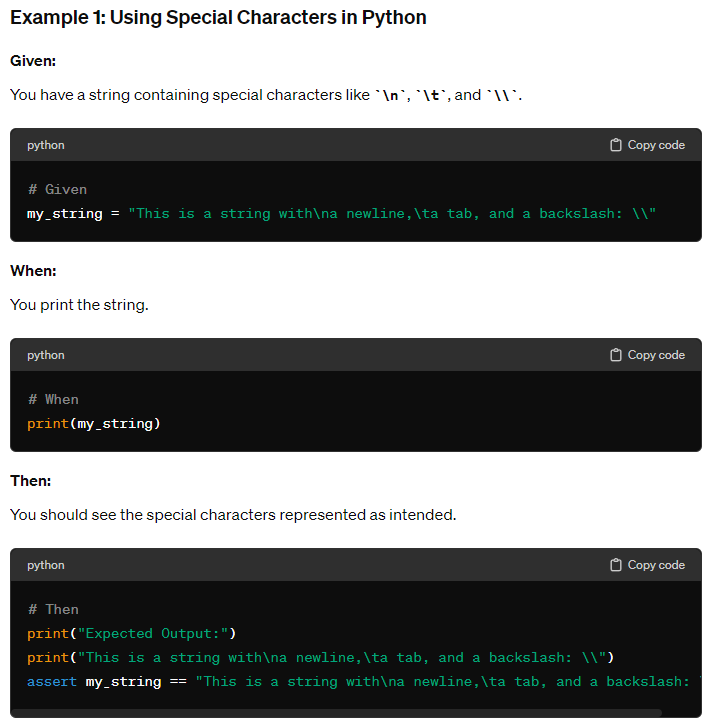
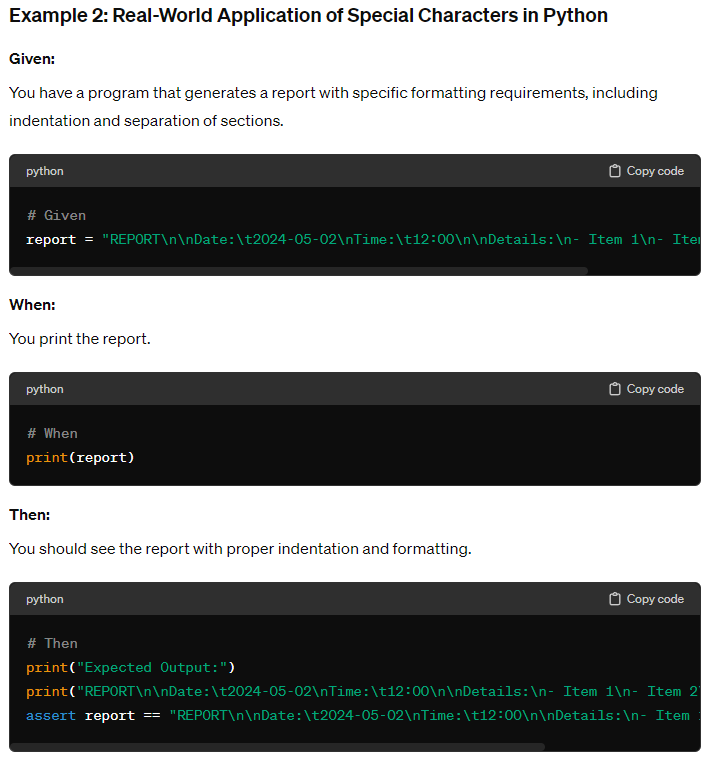
And we can always test the code in our web editors: 💻 Python / 💻 JavaScript / 💻 Groovy / 💻 C#

To get an assignment to learn from:
Can you make a assignment for me to solve?
It has to be about using Special Characters such as '\n', '\t', and '\\'.
The assignment needs to be written in Python as comments.
Use only given, when, and then comments. No code.

And sometimes you might need to start a new session.
Sometimes the AI makes a mistake like writing Given, Then, When (wrong order) and sometimes starting a new session makes it go away.
And you can get AI to solve it for you:
Can you please solve this assignment for me in Python code?
--
# Given a string containing multiple lines of text separated by '\n' characters,
# where some words are separated by '\t' characters,
# and some backslashes are used to escape special characters.
# When the string is given as input,
# Then write a Python program to perform the following tasks:
# 1. Split the string into individual lines using the '\n' character as the delimiter.
# 2. For each line, split the line into words using the '\t' character as the delimiter.
# 3. If a word contains the sequence '\\', replace it with a single backslash character '\'.
# 4. Print each word on a new line.
# For example:
# Given input string:
# "Hello\tworld!\nThis\tis\tan\texample\nString\twith\\tSpecial\tCharacters\n"
# The output should be:
# Hello
# world!
# This
# is
# an
# example
# String
# with\tSpecial
# Characters
--
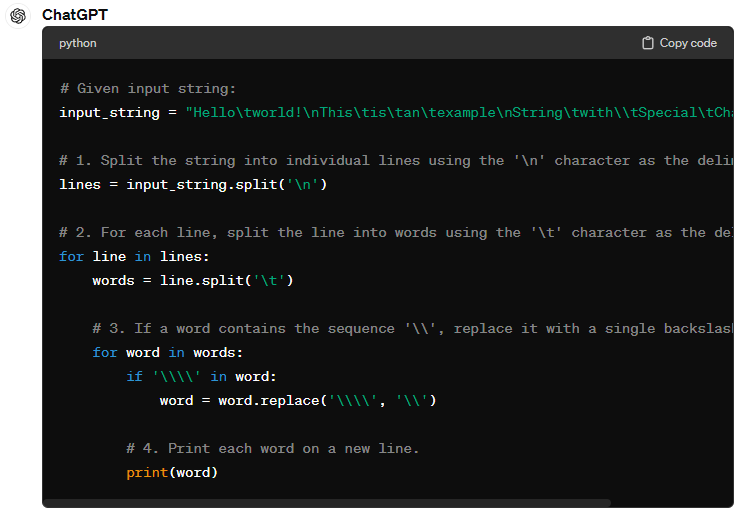
And we can run it in our web editor:
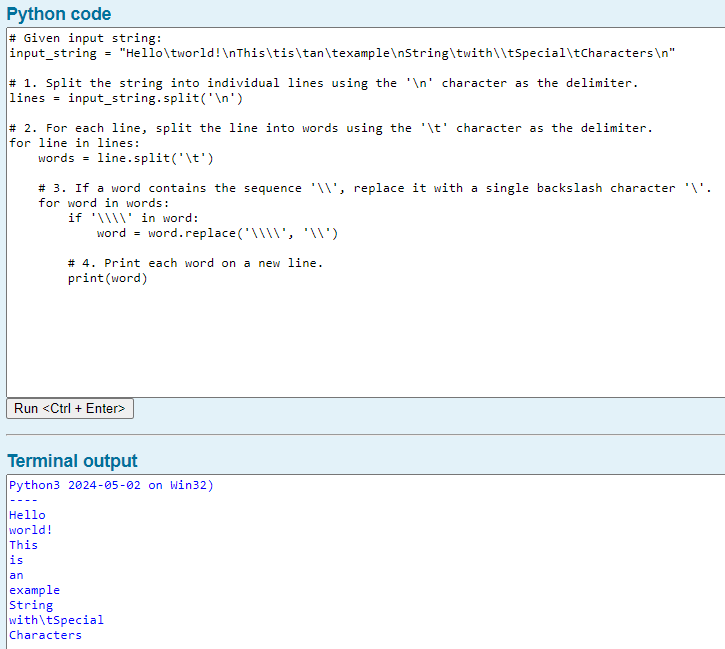
You can ask for a new assignment – as many times as you want.
You can make the AI solve it for you.
You can make the AI help you solve it.
You can solve it yourself.
Limitless learning with a 24/7 tutor!!!
Congratulations – Lesson complete!
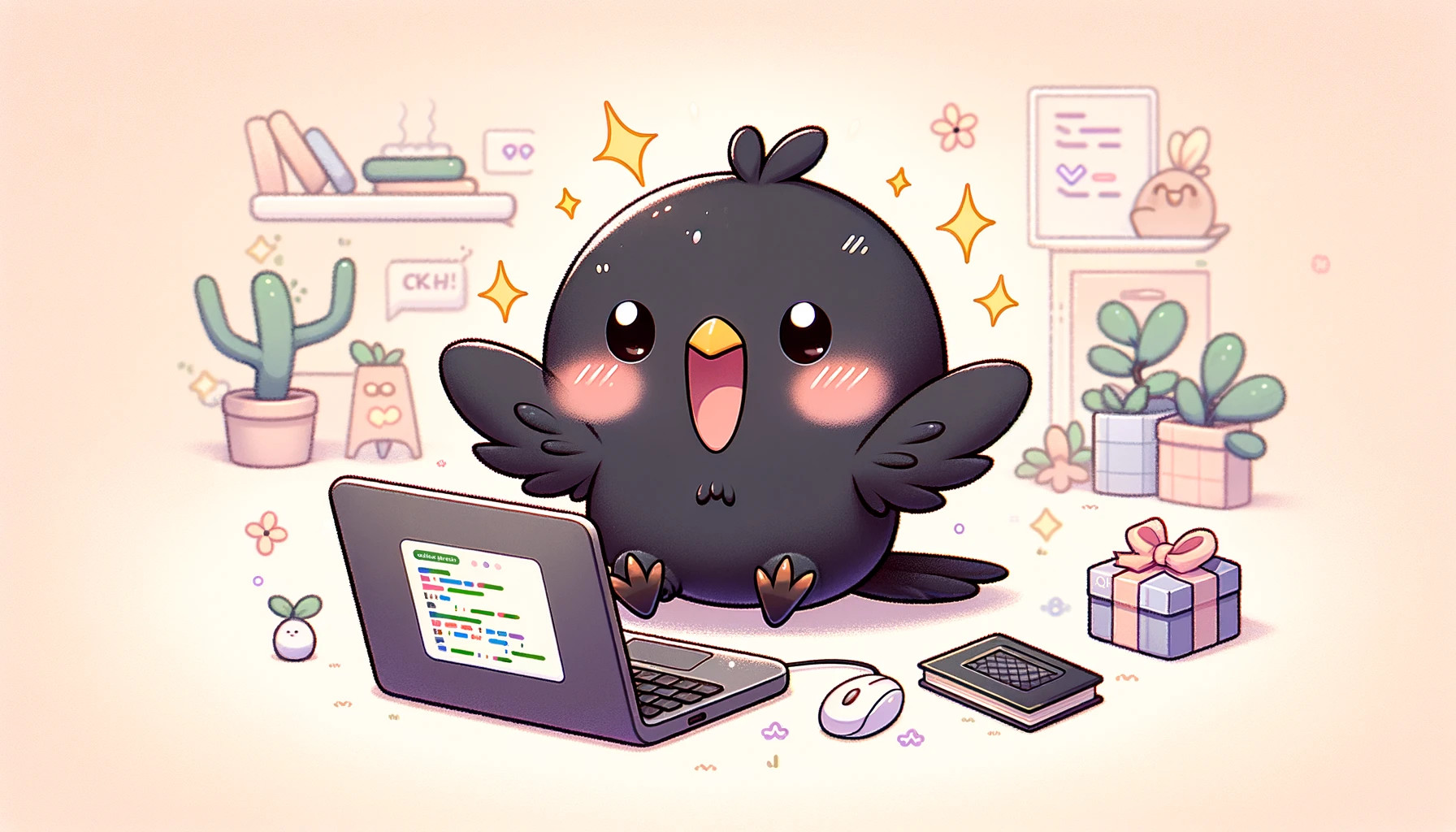