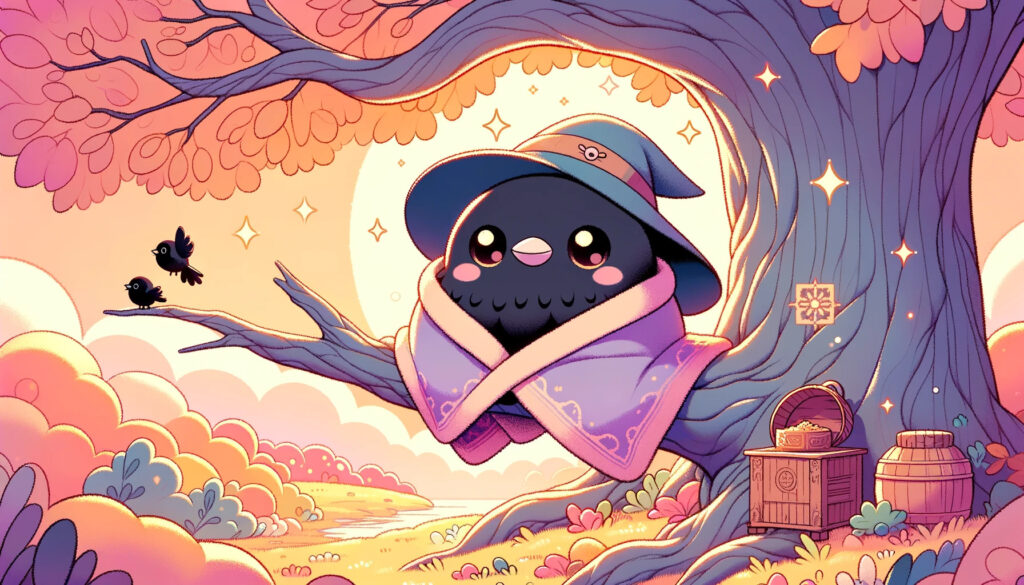
So, you’ve got this awesome idea to dive into the world of coding? That’s seriously awesome! π
Now, I know there’s this fear floating around that programming might become obsolete with all this talk about AI, but trust me – that’s not the case at all! Sure, some parts of programming might change, but that’s just part of the journey.
Back in the old days, programming languages were super specific to hardware. But guess what? Today, they’re becoming more abstract, letting us describe processes and system behaviors in a whole new way!
I’m super confident that the programming languages of the future will be like our own personal translators, helping us communicate exactly what we want. And guess what? We’re gonna dive into the basics together, almost like learning new words to describe really cool stuff! π
Selecting a tool
Please use a web-editor for any language that you want:
Web editor: π» Python / π» JavaScript / π» Groovy / π» C#
I’ve got four awesome code examples for each language above. They’re all kinda similar, so just pick one and dive in! And remember, you can always expand your horizons later on when you’re ready for more!
Writing a comment
Let’s start with your first program.
Let’s kick things off with your very first program, shall we?
We’re gonna start by writing some comments in the code. Comments are like little notes that the computer ignores, but we programmers can see them and get extra info.
I prefer to write code split into 3 phases with comments, so it is like building with Lego:
- Given you have these Lego pieces
- When you put them together in a specific way.
- Then you should get a specific figure.
Simple, right?
How to write a comment:
# This is a comment in Python
// This is a comment in Python
// This is a comment in Python
// This is a comment in Python
As you can see, it’s only Python who uses #, while the other use // for comments.
So, for the first program:
# Given name and a greeting is defined (you can use any name)
name = "Bartek"
greeting = "Hello"
#When the greeting, a space, and the name is combined into the text.
text = greeting + " " + name
# Then the text can be printed as output and be verified by us
print(text)
// Given name and a greeting is defined (you can use any name)
const name = "Bartek";
const greeting = "Hello";
// When the greeting, a space, and the name is combined into the text.
const text = greeting + " " + name;
// Then the text can be printed as output and be verified by us
console.log(text);
// Given name and a greeting is defined (you can use any name)
def name = "Bartek"
def greeting = "Hello"
// When the greeting, a space, and the name is combined into the text.
def text = greeting + " " + name
// Then the text can be printed as output and be verified by us
println(text)
using System;
using System.Diagnostics;
public class Program
{
public static void Main()
{
// Given name and a greeting is defined (you can use any name)
var name = "Bartek";
var greeting = "Hello";
// When the greeting, a space, and the name is combined into the text.
var text = greeting + " " + name;
// Then the text can be printed as output and be verified by us
Console.WriteLine(text);
}
}
Now, I’ve got a little exercise for you: type this code into your web editor of choice:
π» Python / π» JavaScript / π» Groovy / π» C#
If it doesn’t work, no worries! Just use the copy-paste method I’ve included below.
Then, hit run and see the magic happen!
The result of the program should give you a friendly “Hello Bartek,” but feel free to change it up with your own name or different greetings!
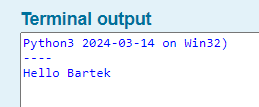
Conclusion
Congratulations – you’ve just written your first program! π Let’s keep this coding adventure going! πͺβ¨
Congratulations – Lesson complete!
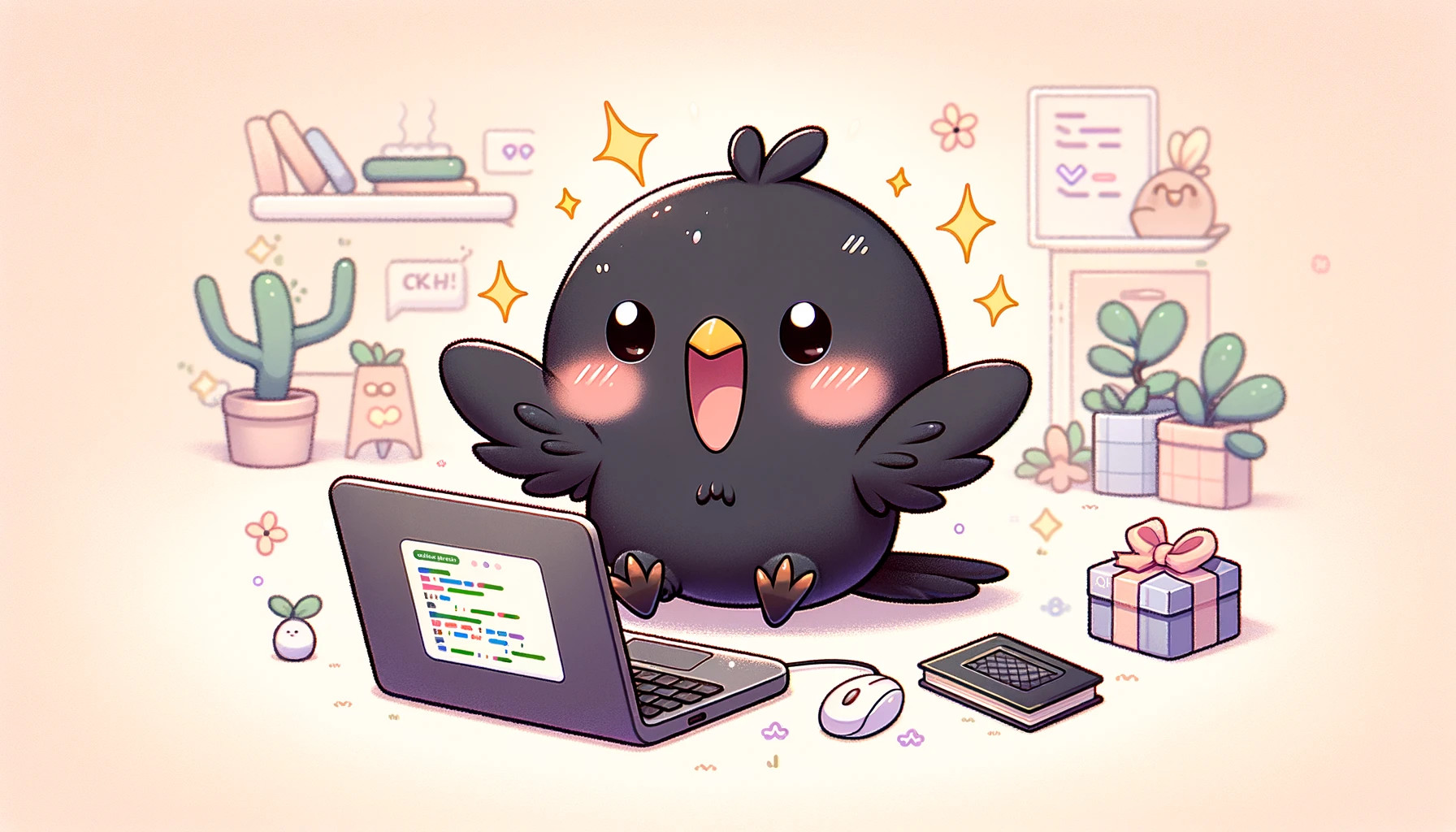